일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- TortoiseSVN
- java
- svn
- Android Studio
- 특징
- 자바
- commit
- Class
- terms
- cherrypick
- IntelliJ
- intellij 연동
- sourcetree
- constructor
- 캡슐화
- gradle
- Checkout
- IntelliJ IDEA Community
- 생성자
- VCS
- error
- git
- 문법
- SSL
- Android
- Subversion
- 상속
- install
- Branch
- syntax
Archives
- Today
- Total
Jay's Developer Note
[JAVA] Class - 1(클래스) 본문
728x90
클래스(Class)
Class 는 같은 모양을 찍어낼 수 있는 거푸집이라고 생각하면 된다.
new 를 통해 Class 를 생성하게 되면 같은 속성, 같은 메소드를 가진 객체 변수를 무한대로 찍어낼 수 있다.
구조
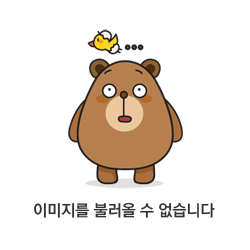
// 클래스 선언부
class ClassName {
// 클래스 멤버
// 생성자
public ClassName() { }
// 멤버 변수
private String classVariable;
// 메소드
public void setClassVariable(String classVariable) {
this.classVariable = classVariable;
}
public String getClassVariable() {
return this.classVariable;
}
// 내부 클래스
private class InnerClass {
// 클래스 멤버
// 생성자
// 멤버 변수
// 메소드
// 내부 클래스
}
}
사용방법
// 클래스 선언
class Car {
// 생성자 : Constructor
public Car() {
System.out.println("Constructor");
}
// 멤버 변수
private int maxSpeed;
// 메소드
public void setMaxSpeed(int maxSpeed) {
this.maxSpeed = maxSpeed;
}
public String getMaxSpeed() {
return this.maxSpeed;
}
}
class Main {
// new 와 생성자를 이용해 Class 를 선언
Car mCar = new Car();
// . 을 사용하면 해당 Class 안에 있는 접근 가능한 변수, 메소드를 사용할 수 있다.
mCar.setMaxSpeed(100);
System.out.printlng(mCar.getMaxSpeed);
}
new 와 생성자를 이용해 참조형 Car 변수를 생성한다.
(참조형 변수에 관한 내용 https://fall-in-it.tistory.com/25?category=998022)
생성자는 따로 만들지 않아도 기본적으로 존재한다.
728x90
'JAVA' 카테고리의 다른 글
[JAVA] Class - 3(멤버 변수) (0) | 2022.03.11 |
---|---|
[JAVA] Class - 2(생성자) (0) | 2022.02.25 |
[JAVA] Intellij 에서 JAVA 실행하기 (0) | 2022.02.18 |
[JAVA] 자바 언어 기본 문법 - 6(분기문) (0) | 2022.02.13 |
[JAVA] 자바 언어 기본 문법 - 5(반복문) (0) | 2022.02.12 |